imageflip
(PHP 5 >= 5.5.0, PHP 7)
imageflip — Flips an image using a given mode
Описание
$image
, int $mode
)
Flips the image
image using the given
mode
.
Список параметров
-
image
-
Ресурс изображения, полученный одной из функций создания изображений, например, такой как imagecreatetruecolor().
-
mode
-
Flip mode, this can be one of the
IMG_FLIP_*
constants:Constant Meaning IMG_FLIP_HORIZONTAL
Flips the image horizontally. IMG_FLIP_VERTICAL
Flips the image vertically. IMG_FLIP_BOTH
Flips the image both horizontally and vertically.
Возвращаемые значения
Возвращает TRUE
в случае успешного завершения или FALSE
в случае возникновения ошибки.
Примеры
Пример #1 Flips an image vertically
This example uses the IMG_FLIP_VERTICAL
constant.
<?php
// File
$filename = 'phplogo.png';
// Content type
header('Content-type: image/png');
// Load
$im = imagecreatefrompng($filename);
// Flip it vertically
imageflip($im, IMG_FLIP_VERTICAL);
// Output
imagejpeg($im);
imagedestroy($im);
?>
Результатом выполнения данного примера будет что-то подобное:
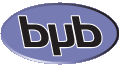
Пример #2 Flips the image horizontally
This example uses the IMG_FLIP_HORIZONTAL
constant.
<?php
// File
$filename = 'phplogo.png';
// Content type
header('Content-type: image/png');
// Load
$im = imagecreatefrompng($filename);
// Flip it horizontally
imageflip($im, IMG_FLIP_HORIZONTAL);
// Output
imagejpeg($im);
imagedestroy($im);
?>
Результатом выполнения данного примера будет что-то подобное:
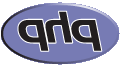
- PHP Руководство
- Функции по категориям
- Индекс функций
- Справочник функций
- Обработка и генерация изображений
- Обработка изображений и GD
- gd_info
- getimagesize
- getimagesizefromstring
- image_type_to_extension
- image_type_to_mime_type
- image2wbmp
- imageaffine
- imageaffinematrixconcat
- imageaffinematrixget
- imagealphablending
- imageantialias
- imagearc
- imagechar
- imagecharup
- imagecolorallocate
- imagecolorallocatealpha
- imagecolorat
- imagecolorclosest
- imagecolorclosestalpha
- imagecolorclosesthwb
- imagecolordeallocate
- imagecolorexact
- imagecolorexactalpha
- imagecolormatch
- imagecolorresolve
- imagecolorresolvealpha
- imagecolorset
- imagecolorsforindex
- imagecolorstotal
- imagecolortransparent
- imageconvolution
- imagecopy
- imagecopymerge
- imagecopymergegray
- imagecopyresampled
- imagecopyresized
- imagecreate
- imagecreatefromgd2
- imagecreatefromgd2part
- imagecreatefromgd
- imagecreatefromgif
- imagecreatefromjpeg
- imagecreatefrompng
- imagecreatefromstring
- imagecreatefromwbmp
- imagecreatefromwebp
- imagecreatefromxbm
- imagecreatefromxpm
- imagecreatetruecolor
- imagecrop
- imagecropauto
- imagedashedline
- imagedestroy
- imageellipse
- imagefill
- imagefilledarc
- imagefilledellipse
- imagefilledpolygon
- imagefilledrectangle
- imagefilltoborder
- imagefilter
- imageflip
- imagefontheight
- imagefontwidth
- imageftbbox
- imagefttext
- imagegammacorrect
- imagegd2
- imagegd
- imagegif
- imagegrabscreen
- imagegrabwindow
- imageinterlace
- imageistruecolor
- imagejpeg
- imagelayereffect
- imageline
- imageloadfont
- imagepalettecopy
- imagepalettetotruecolor
- imagepng
- imagepolygon
- imagepsbbox
- imagepsencodefont
- imagepsextendfont
- imagepsfreefont
- imagepsloadfont
- imagepsslantfont
- imagepstext
- imagerectangle
- imagerotate
- imagesavealpha
- imagescale
- imagesetbrush
- imagesetinterpolation
- imagesetpixel
- imagesetstyle
- imagesetthickness
- imagesettile
- imagestring
- imagestringup
- imagesx
- imagesy
- imagetruecolortopalette
- imagettfbbox
- imagettftext
- imagetypes
- imagewbmp
- imagewebp
- imagexbm
- iptcembed
- iptcparse
- jpeg2wbmp
- png2wbmp
Коментарии
If you're using PHP < 5.5 you can use this code to add the same functionality. If you pass the wrong $mode in it will silently fail. You might want to add your own error handling for this case.
<?php
if (!function_exists('imageflip')) {
define('IMG_FLIP_HORIZONTAL', 0);
define('IMG_FLIP_VERTICAL', 1);
define('IMG_FLIP_BOTH', 2);
function imageflip($image, $mode) {
switch ($mode) {
case IMG_FLIP_HORIZONTAL: {
$max_x = imagesx($image) - 1;
$half_x = $max_x / 2;
$sy = imagesy($image);
$temp_image = imageistruecolor($image)? imagecreatetruecolor(1, $sy): imagecreate(1, $sy);
for ($x = 0; $x < $half_x; ++$x) {
imagecopy($temp_image, $image, 0, 0, $x, 0, 1, $sy);
imagecopy($image, $image, $x, 0, $max_x - $x, 0, 1, $sy);
imagecopy($image, $temp_image, $max_x - $x, 0, 0, 0, 1, $sy);
}
break;
}
case IMG_FLIP_VERTICAL: {
$sx = imagesx($image);
$max_y = imagesy($image) - 1;
$half_y = $max_y / 2;
$temp_image = imageistruecolor($image)? imagecreatetruecolor($sx, 1): imagecreate($sx, 1);
for ($y = 0; $y < $half_y; ++$y) {
imagecopy($temp_image, $image, 0, 0, 0, $y, $sx, 1);
imagecopy($image, $image, 0, $y, 0, $max_y - $y, $sx, 1);
imagecopy($image, $temp_image, 0, $max_y - $y, 0, 0, $sx, 1);
}
break;
}
case IMG_FLIP_BOTH: {
$sx = imagesx($image);
$sy = imagesy($image);
$temp_image = imagerotate($image, 180, 0);
imagecopy($image, $temp_image, 0, 0, 0, 0, $sx, $sy);
break;
}
default: {
return;
}
}
imagedestroy($temp_image);
}
}
?>