imagecolorallocatealpha
(PHP 4 >= 4.3.2, PHP 5)
imagecolorallocatealpha — Allocate a color for an image
Description
$image
, int $red
, int $green
, int $blue
, int $alpha
)
imagecolorallocatealpha() behaves identically to
imagecolorallocate() with the addition of the transparency
parameter alpha
.
Parameters
-
image
-
An image resource, returned by one of the image creation functions, such as imagecreatetruecolor().
-
red
-
Value of red component.
-
green
-
Value of green component.
-
blue
-
Value of blue component.
-
alpha
-
A value between 0 and 127. 0 indicates completely opaque while 127 indicates completely transparent.
red
, green
and blue
parameters are integers
between 0 and 255 or hexadecimals between 0x00 and 0xFF.
Return Values
A color identifier or FALSE
if the allocation failed.
This function may
return Boolean FALSE
, but may also return a non-Boolean value which
evaluates to FALSE
. Please read the section on Booleans for more
information. Use the ===
operator for testing the return value of this
function.
Changelog
Version | Description |
---|---|
5.1.3 |
Returns FALSE if the allocation failed. Previously
-1 was returned.
|
Examples
Example #1 Example of using imagecolorallocatealpha()
<?php
$size = 300;
$image=imagecreatetruecolor($size, $size);
// something to get a white background with black border
$back = imagecolorallocate($image, 255, 255, 255);
$border = imagecolorallocate($image, 0, 0, 0);
imagefilledrectangle($image, 0, 0, $size - 1, $size - 1, $back);
imagerectangle($image, 0, 0, $size - 1, $size - 1, $border);
$yellow_x = 100;
$yellow_y = 75;
$red_x = 120;
$red_y = 165;
$blue_x = 187;
$blue_y = 125;
$radius = 150;
// allocate colors with alpha values
$yellow = imagecolorallocatealpha($image, 255, 255, 0, 75);
$red = imagecolorallocatealpha($image, 255, 0, 0, 75);
$blue = imagecolorallocatealpha($image, 0, 0, 255, 75);
// drawing 3 overlapped circle
imagefilledellipse($image, $yellow_x, $yellow_y, $radius, $radius, $yellow);
imagefilledellipse($image, $red_x, $red_y, $radius, $radius, $red);
imagefilledellipse($image, $blue_x, $blue_y, $radius, $radius, $blue);
// don't forget to output a correct header!
header('Content-Type: image/png');
// and finally, output the result
imagepng($image);
imagedestroy($image);
?>
The above example will output something similar to:
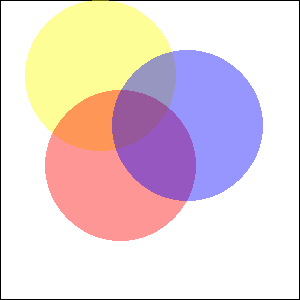
Notes
Note: This function requires GD 2.0.1 or later (2.0.28 or later is recommended).
See Also
- imagecolorallocate() - Allocate a color for an image
- imagecolordeallocate() - De-allocate a color for an image
- PHP Руководство
- Функции по категориям
- Индекс функций
- Справочник функций
- Обработка и генерация изображений
- Обработка изображений и GD
- gd_info
- getimagesize
- getimagesizefromstring
- image_type_to_extension
- image_type_to_mime_type
- image2wbmp
- imageaffine
- imageaffinematrixconcat
- imageaffinematrixget
- imagealphablending
- imageantialias
- imagearc
- imagechar
- imagecharup
- imagecolorallocate
- imagecolorallocatealpha
- imagecolorat
- imagecolorclosest
- imagecolorclosestalpha
- imagecolorclosesthwb
- imagecolordeallocate
- imagecolorexact
- imagecolorexactalpha
- imagecolormatch
- imagecolorresolve
- imagecolorresolvealpha
- imagecolorset
- imagecolorsforindex
- imagecolorstotal
- imagecolortransparent
- imageconvolution
- imagecopy
- imagecopymerge
- imagecopymergegray
- imagecopyresampled
- imagecopyresized
- imagecreate
- imagecreatefromgd2
- imagecreatefromgd2part
- imagecreatefromgd
- imagecreatefromgif
- imagecreatefromjpeg
- imagecreatefrompng
- imagecreatefromstring
- imagecreatefromwbmp
- imagecreatefromwebp
- imagecreatefromxbm
- imagecreatefromxpm
- imagecreatetruecolor
- imagecrop
- imagecropauto
- imagedashedline
- imagedestroy
- imageellipse
- imagefill
- imagefilledarc
- imagefilledellipse
- imagefilledpolygon
- imagefilledrectangle
- imagefilltoborder
- imagefilter
- imageflip
- imagefontheight
- imagefontwidth
- imageftbbox
- imagefttext
- imagegammacorrect
- imagegd2
- imagegd
- imagegif
- imagegrabscreen
- imagegrabwindow
- imageinterlace
- imageistruecolor
- imagejpeg
- imagelayereffect
- imageline
- imageloadfont
- imagepalettecopy
- imagepalettetotruecolor
- imagepng
- imagepolygon
- imagepsbbox
- imagepsencodefont
- imagepsextendfont
- imagepsfreefont
- imagepsloadfont
- imagepsslantfont
- imagepstext
- imagerectangle
- imagerotate
- imagesavealpha
- imagescale
- imagesetbrush
- imagesetinterpolation
- imagesetpixel
- imagesetstyle
- imagesetthickness
- imagesettile
- imagestring
- imagestringup
- imagesx
- imagesy
- imagetruecolortopalette
- imagettfbbox
- imagettftext
- imagetypes
- imagewbmp
- imagewebp
- imagexbm
- iptcembed
- iptcparse
- jpeg2wbmp
- png2wbmp
Коментарии
If you only wish to extract the alpha value for a color, you can simply extract it like so:
<?php
$color = imagecolorat($im, 50, 50);
$alpha = $color >> 24;
?>
It actually shifts off the first 24 bits (where 8x3 are used for each color), and returns the remaining 7 allocated bits (commonly used for alpha)
If you need to calculate the integer representation of a color with an alpha channel, without initialising an image and using the imagecolorallocatealpha function. Then this function might be of some help:
<?php
function alphaColor($hexColor,$alpha)
{
return bindec(decbin($alpha).decbin(hexdec($hexColor));
}
echo alphaColor("FFFFFF",127);
?>
When working with transparency, avoid imagecreate() and use imagecreatetruecolor() instead. Transparency effects may not work as expected within a palette-based image.